ใน Python coroutine เป็นฟังก์ชันที่สามารถทำงานแบบไม่พร้อมกันได้ coroutine จะถูกเรียกใช้งานโดย asyncio.run() หรือ asyncio.run_until_complete()
task เป็นตัวแทนของ coroutine ที่ถูกกำหนดเวลาให้ทำงานแบบไม่พร้อมกัน task จะถูกสร้างขึ้นโดย asyncio.create_task()
ในบทความนี้ เราจะเรียนรู้วิธีสร้าง coroutine task in python อย่างละเอียดยิ่งขึ้น
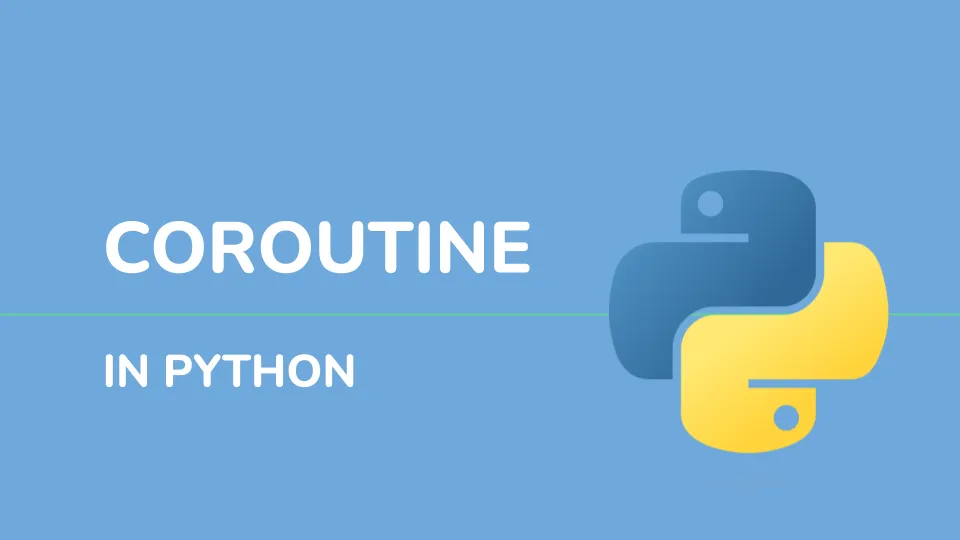
การสร้าง coroutine
ในการสร้าง coroutine เราต้องสร้างฟังก์ชันโดยใช้คำกริยา async def
ฟังก์ชัน coroutine แตกต่างจากฟังก์ชันปกติตรงที่สามารถใช้คำกริยา await เพื่อรอให้การดำเนินการอื่นเสร็จสิ้น
ตัวอย่างเช่น ต่อไปนี้เป็น coroutine ที่พิมพ์ข้อความ “Hello, world!” และรอ 1 วินาที
async def hello_world():
print("Hello, world!")
await asyncio.sleep(1)
การสร้าง task
ในการสร้าง task เราใช้ฟังก์ชัน asyncio.create_task()
ฟังก์ชันนี้รับ coroutine เป็นตัวดำเนินการและสร้าง task ใหม่
ตัวอย่างเช่น ต่อไปนี้เป็นการสร้าง task จาก coroutine hello_world()
import asyncio
async def main():
task = asyncio.create_task(hello_world())
await task
asyncio.run(main())
คุณสมบัติของ task
task มีคุณสมบัติหลายประการที่สามารถใช้เพื่อจัดการกับ task เหล่านั้นได้
- task.done(): ตรวจสอบว่า task เสร็จสิ้นหรือยัง
- task.result(): ดึงผลลัพธ์ของ task
- task.cancel(): ยกเลิก task
ตัวอย่างเช่น ต่อไปนี้เป็นตัวอย่างการใช้คุณสมบัติของ task
import asyncio
async def main():
task = asyncio.create_task(hello_world())
print("Task is created")
if task.done():
print("Task is already finished")
else:
print("Task is not finished yet")
print("Result:", task.result())
# Cancel task
task.cancel()
asyncio.run(main())
ผลลัพธ์:
Task is created
Task is not finished yet
Hello, world!
Result: None
ตัวอย่างการใช้งาน
ต่อไปนี้เป็นตัวอย่างการใช้งาน coroutine task
- การดาวน์โหลดไฟล์
เราสามารถใช้ coroutine task เพื่อดาวน์โหลดไฟล์แบบไม่พร้อมกันได้
import asyncio
async def download_file(url):
with open(url, "wb") as f:
response = await requests.get(url)
f.write(response.content)
async def main():
tasks = []
for url in ["https://example.com/file1.txt", "https://example.com/file2.txt"]:
tasks.append(asyncio.create_task(download_file(url)))
await asyncio.gather(*tasks)
asyncio.run(main())
- การประมวลผลข้อมูล
เราสามารถใช้ coroutine task เพื่อประมวลผลข้อมูลแบบไม่พร้อมกันได้
import asyncio
async def process_data(data):
print(f"Processing data: {data}")
await asyncio.sleep(1)
async def main():
tasks = []
for data in ["data1", "data2", "data3"]:
tasks.append(asyncio.create_task(process_data(data)))
await asyncio.gather(*tasks)
asyncio.run(main())
สรุปท้ายบทความ
ในบทความนี้ เราได้เรียนรู้วิธีสร้าง coroutine task in python อย่างละเอียดยิ่งขึ้น
- ในการสร้าง coroutine เราต้องสร้างฟังก์ชันโดยใช้คำกริยา async def
- ในการสร้าง task เราใช้ฟังก์ชัน asyncio.create_task()
- task มีคุณสมบัติหลายประการที่สามารถใช้เพื่อจัดการกับ task เหล่านั้นได้
หวังว่าบทความนี้จะเป็นประโยชน์นะครับ